Back to Projects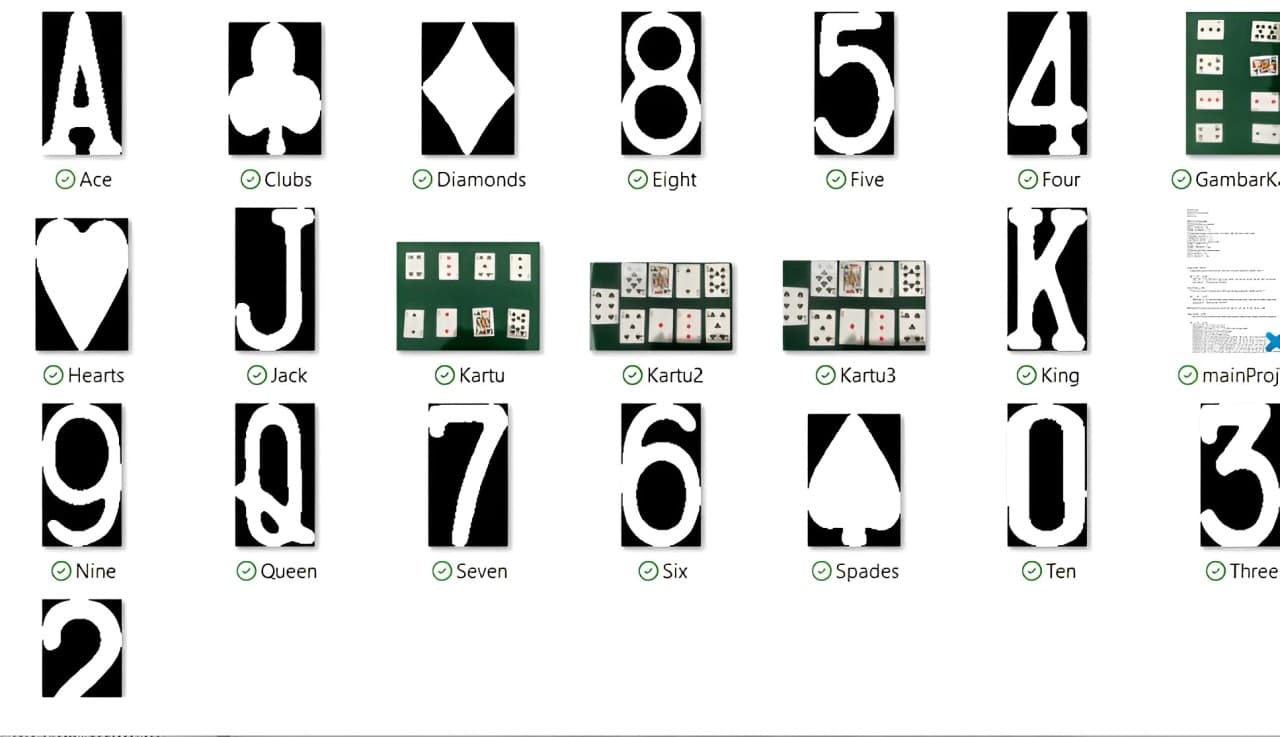
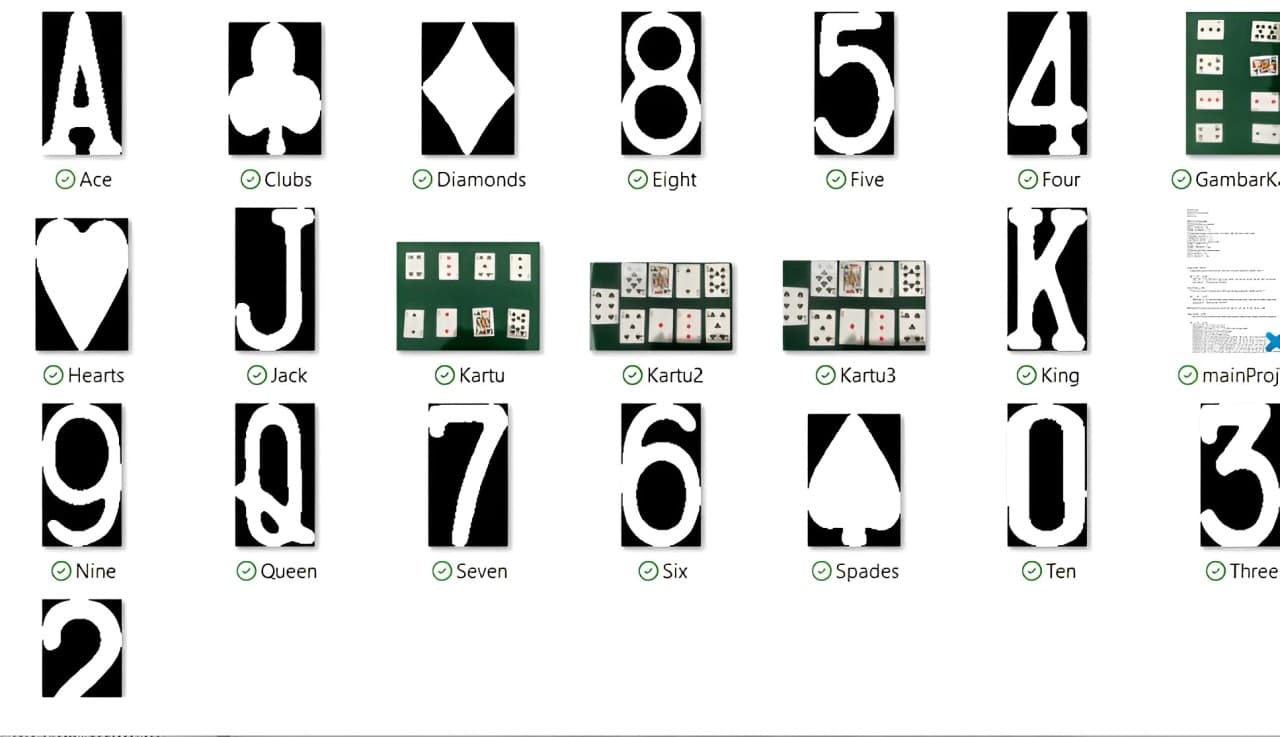
Detection Card Blackjack
Game DevelopmentOctober 2023 - December 2023View Source Code
Technologies Used
PythonOpenCVNumPyTkinter
About the Project
Detection Card Blackjack is an innovative card game that combines computer vision with the classic game of Blackjack. Using OpenCV, the game can detect and recognize playing cards in real-time through a webcam, creating an interactive gaming experience that bridges physical cards with digital gameplay.
Key Features
- Real-time card detection using OpenCV
- Card value recognition and scoring system
- User-friendly interface built with Tkinter
- Standard Blackjack game rules implementation
- Webcam integration for card capture
Implementation Details
The project is implemented in Python and utilizes OpenCV for image processing and card detection. The game follows these main steps:
- Card Detection: Using contour detection to identify card shapes
- Corner Detection: Identifying card corners to determine values
- Value Recognition: Processing detected corners to determine card values
- Game Logic: Implementing standard Blackjack rules
- User Interface: Creating an intuitive UI with Tkinter
Code Snippet
def find_cards(frame):
# Convert to grayscale
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# Find contours
contours, _ = cv2.findContours(
gray, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE
)
# Process each contour
for contour in contours:
# Get card value
card_value = process_card(contour)
if card_value:
cards.append(card_value)
return cards
Requirements
- Python 3.x
- OpenCV (cv2)
- NumPy
- Tkinter
- Webcam or camera device